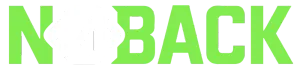
Build Secure, Scalable Backends in Minutes on AWS
noback is an open-source framework that makes serverless developments a breeze, with automated deployments, cost-optimized, and several layers of security built right in
import { Security, DB, S3 } from 'noback-layer'
export async function handler(event) {
const { token, userId } = Security.validateHeader(
event.headers.Authorization
)
try {
const user = JSON.parse(event.body)
// Store in DynamoDB
await DB.user.save({
...user,
country: event.headers['CloudFront-Viewer-Country']
})
// Update S3 cache
await S3.updateCache({
userId,
data: { lastActivity: new Date() }
})
return Security.corsResponse(200, { token })
} catch (err) {
return Security.corsResponse(500, err)
}
}
Why noback?
Rapid Development
- Lambda Layers for dependencies & common functionality
- Infrastructure as Code templates for one-step deployments
- Minimal boilerplate, highest productivity
- Preconfigured settings for most AWS services
- Zero server maintenance
Cost Optimization
- Serverless-based approach - pay only for actual usage
- DynamoDB single-table design for efficient reads/writes
- Multiple layers of cache for cost reduction
- Automatic resource scaling based on workload
- Cost monitoring and optimization recommendations
Effortless Scalability
- Scale seamlessly leveraging AWS capabilities
- Scalability from the first minute
- Built on API Gateway, Lambda, S3, DynamoDB, and EventBridge
- Caches and geodistribution capabilities
- Automatic horizontal scaling
Advanced Security
- ACL tied to Cognito roles for granular access
- Access control patterns defined by infrastructure as code
- JWT fingerprinting for enhanced security
- Proactive suspicious activity detection
- WAF integration with geofencing capabilities
Performance
- Optimized DynamoDB operations with single-table design
- Configurable Lambda RAM for specific function requirements
- Multi-layer caching strategy (API Gateway, CloudFront, S3)
- Performance profiling and monitoring tools
- Cold start optimization techniques
Observability
- Comprehensive CloudWatch metrics and alarms
- End-to-end request tracing with AWS X-Ray
- Detailed logging and error tracking
- Custom dashboards for monitoring
- Resource utilization insights
How does noback compare?
noback combines the best of AWS with top-notch security, minus the overhead of fixed costs.
Feature | noback | Supabase | 8base | Firebase |
---|---|---|---|---|
Core Infrastructure | AWS (100% serverless) | Managed PostgreSQL | Aurora/MySQL (partial serverless) | Google Cloud (serverless) |
Auth & Roles | Cognito + SSO + Fingerprinting | GoTrue (basic JWT) | Auth0 or Custom | Firebase Auth + Custom Roles |
Dev Speed | High (Layers + IaC) | Medium (SQL + APIs) | Medium (GraphQL + UI) | High (SDK + UI Tools) |
Advanced Security | Yes (WAF + geofencing + IP lists) | Limited (manual config) | Depends on manual config | Basic (App-level security) |
Cost Model | Pay-per-use (fully serverless) | Hosted plans / managed DB | Mixed (DB cost + additional services) | Pay-per-use + quotas |
Technical Features
Lambda Layers for Fast Development
Common layers for security, SQS (queues), S3 (storage), DynamoDB, EventBridge, etc.
import { Security, Data, parseJwt } from 'noback-layer'
export async function handler(event) {
// Your code here
}
Scalability & Cost
Enterprise-grade scalability with cost-effective solutions.
- 1,000+ req/sec out of the box
- 100% serverless pay-per-use model
- DynamoDB single-table design with several projections
- CloudFront + S3 for static content delivery (frontend)
Security
Enterprise-grade security features built-in.
- JWT fingerprinting (IP + user-agent)
- ACL at token level (Cognito roles)
- WAF (country filters, IP white/blacklists)
- Integration with Cognito, IAM Roles, geofencing
Observability & Monitoring
Comprehensive monitoring and observability tools.
- X-Ray everywhere
- Custom metrics in CloudWatch
- Example dashboard screenshots
Professional Services & noback Foundation
noback Foundation
Open-source Mission
- Ensuring continuity and evolution of the framework
- Allow every developer in the world to run high standard backends to foster innovation
- Building a strong, collaborative open-source community
Open Source Project
Contribute to noback's development and help shape the future of serverless.
View on GitHubServices & Consulting
-
AWS Serverless Migrations
Expert guidance in migrating your infrastructure to serverless architecture
-
Security Audits
Comprehensive security reviews including WAF, ACL, and more
-
Custom Development
Tailored solutions built on noback framework
Ticket-based Model 🤩
Transparent budgets open to the community
Roadmap & Community
Roadmap
AI-based Entity Generation
Introducing simpleback: AI-powered entity and schema generation for faster development.
S3 System Cache
Implement S3-based object storage system to scale beyond Lambda function size limits and optimize performance.
Multi-region Support
Seamless deployment across multiple AWS regions with automatic failover.
Frequently Asked Questions
noback follows a fully serverless architecture, meaning you only pay for what you use. The DynamoDB single-table design helps reduce overhead costs.
Note: Some AWS services like WAF may have minimum spending requirements regardless of usage.
While basic AWS knowledge is recommended, noback significantly simplifies many aspects of serverless development. Our framework abstracts away much of the complexity, making it easier to get started.
There are several ways to contribute to noback:
- Submit pull requests on GitHub
- Report issues and bugs
- Share feedback and suggestions
- Help improve documentation
- Share your experience with the community